OCCUtils provides an easy way of exporting a STEP with colored elements:
#include <occutils/ExtendedSTEP.hxx>
#include <occutils/Primitive.hxx>
TopoDS_Shape cube = Primitive::MakeCube(5 /* mm */);
STEP::ExtendedSTEPExporter stepExporter;
stepExporter.AddShapeWithColor(cube, Quantity_NOC_RED);
stepExporter.Write("ColoredCube.step");
The resulting STEP file looks like this:
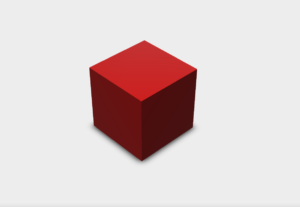
You can also add non-colored shapes:
stepExporter.AddShape(myShape);
Doing it without OCCUtils is possible but might make your life miserable since it’s rather hard to get to work correctly.
Here’s a minimal example:
// Get global application
Handle(TDocStd_Application) application = XCAFApp_Application::GetApplication();
// Create document
Handle(TDocStd_Document) document;
application->NewDocument("MDTV-XCAF", document);
// Get shape & color tools
Handle(XCAFDoc_ShapeTool) shapeTool = XCAFDoc_DocumentTool::ShapeTool(document->Main());
Handle(XCAFDoc_ColorTool) colorTool = XCAFDoc_DocumentTool::ColorTool(document->Main());
// Create shape
// IMPORTANT: DO NOT use shapeTool->AddShape(TopoDS_Shape)!
// This WILL NOT PRESERVE the color!
TDF_Label partLabel = shapeTool->NewShape();
shapeTool->SetShape(partLabel, filletedBody);
//TDF_Label redColor = colorTool->AddColor();
colorTool->SetColor(partLabel, Quantity_NOC_RED, XCAFDoc_ColorGen);
STEPCAFControl_Writer writer;
writer.SetColorMode(true);
writer.Perform(document, "ColoredShape.step");
What colors can you use?
Either define the Quantity_Color
yourself using RGB values (from 0.0
to 1.0
) like this:
Quantity_Color red(1.0 /* R */, 0.0 /* G */, 0.0 /* B */, Quantity_TypeOfColor::Quantity_TOC_RGB);
alternatively you can do the same in the HSL colorspace:
Quantity_Color brownishOrange(1.0 /* H */, 0.5 /* L */, 0.5 /* S */, Quantity_TypeOfColor::Quantity_TOC_HLS);
or you can use the predefined colors in the Quantity_NameOfColor
enum – see Overview of all standard colors available in OpenCASCADE for a table of all available colors.